前言
说明一下springboot2.0.6.RELEASE集成jooq3.10.8以及jooq-codegen-maven3.10.8
目的
简单说明一下jooq的集成和用法
正文
初始化工程
idea初始化工程方法可以参见**springcloud编写用户微服务**
h2
以h2为梨子说明一下
添加依赖
1 | <dependency> |
扩展
这种不需要写版本的是因为父工程为springboot或者用下面那种写法
1 | <parent> |
添加驱动工具
1 | <plugin> |
扩展
1.这是以h2作为梨子,如果要用mysql的话差不过需要修改相关位置
2.maven package打包忽略插件
放在pom驱动配置的configuration标签内
20181030更新:注意这个标签添加之后,无论编译打包甚至直接运行这个插件都不再生成相关东西,如果你现在需要重新运行一遍,请先提交或者保存你做过的修改,然后注释掉这个,运行完之后再加上,然后再将你做的修改重新添加回来
1 | <!-- install 跳过 --> |
3.需要生成相关dao和实体,添加如下配置
1 | <generate> |
4.20181030更新:当h2的数据库类型为内存时,结果jooq-codegen-maven无法生成
1 | <!-- JDBC connection parameters --> |
需要改成文件类型
1 | <!-- JDBC connection parameters --> |
jooq-codegen-maven以上配置生成的目录结构如下
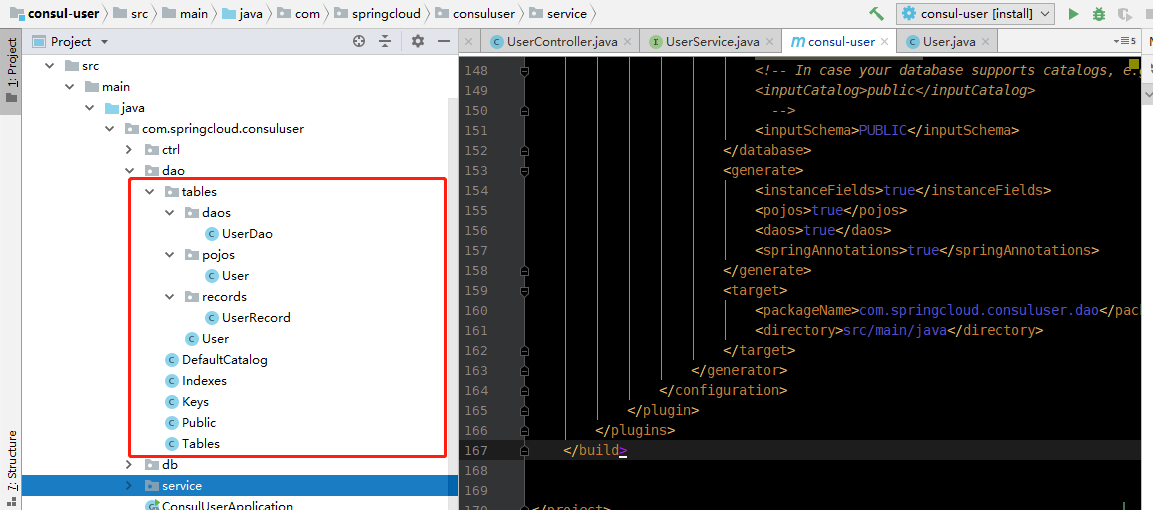
yml配置文件添加相关配置
1 | spring: |
扩展
1.上面的配置中添加initialization-mode之后才会初始化schema.sql和data.sql
2.initialization-mode配置之后的是druid的补充配置,需结合java程序手动初始化或者添加spring.datasource.type=com.alibaba.druid.pool.DruidDataSource自动初始化,不过这种初始化之前我的druid监控页面无法打开
3.h2的控制页面配置如上,如果不加无法访问
4.集成druid,详情见**springboot集成druid**