前言
1.springcloud编写用户微服务
2.springcloud编写电影微服务
3.springcloud集成网关ZUUL
依上面教程,我已经实现了用户,电影微服务以及zuul网关,微服务的设计难点之一在于对原有业务的拆分,
在我看来每个微服务职责要尽可能单一,但是这样同样也带来了一个问题,那就是微服务之间不可避免的一
些交集.例如终端需要查询用户信息和电影信息,这里有两种做法
1.让终端查询用户信息后在查询电影信息
2.网关层查询用户信息和电影信息,聚合后返回给终端
后一种方式显然更好一些,因为他节省了带宽,相较于终端两次请求网关,显然网关两次请求微服务的网络情况更好
目的
利用RXJAVA聚合微服务,这里面其实很多东西可以讨论,关于分布式协议和分布式事务,这次先简单的说明
一下查询聚合,因为查询是幂等操作,不需要事务
正文
工程初始化
可以参见这篇博客**springcloud集成网关ZUUL**
验证:
http://localhost:1051/api/user/user/getAll
http://localhost:1051/api/movie/movie/findOneById?id=2
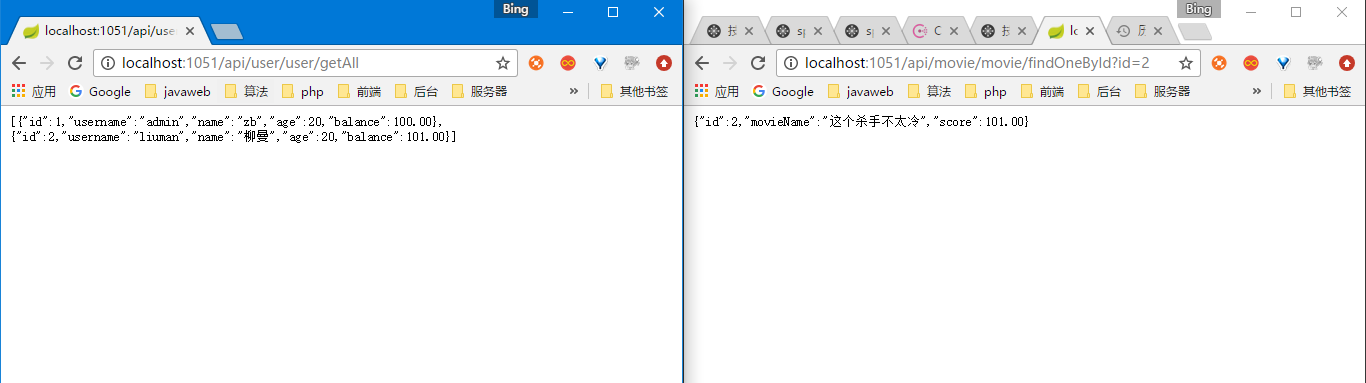
集成feign,添加用户和电影的消费端
可以参见这篇博客**springcloud集成feign**
仿照user创建movie的feign客户端
1 | @FeignClient(name = "consul-movie") |
get多参数写法
1 | 直接写Long id或者直接是User user这种对象,feign依然会用post方式调用,所以会报错接口不支持 |
扩展
20181031更新:zuul上的service中添加hystrix回退并没有执行,如下图,想着service中利用的是
feign实现的,所以给feign加上回退,需要在配置上加上feign.hystrix.enabled=true
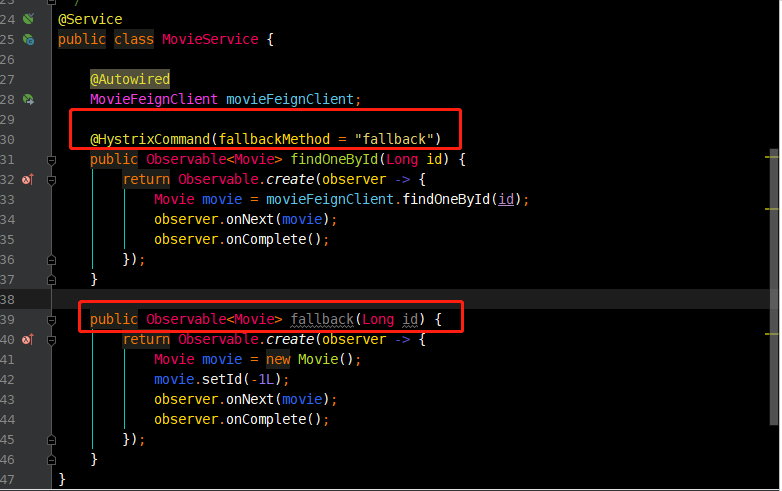
1 | "consul-movie",fallback = MovieFeignClient.MovieFeignClientFallBack.class) (name = |
集成ribbon
添加一个配置类java
1 |
|
添加ribbon调用服务类
1 |
|
新建聚合controller和服务(RXjava)
针对feign实现的service,之前在上面截图中可以发现我在上面加了HystrixCommand没有用,所以去掉了,不知道是不是因为里面是feign实现的,所以下面会有ribbon实现
1 | /** |
控制类
1 | /** |
访问
feign http://localhost:1051/um/getUserAndMovieUseRx?id=1 hystrix有效
rxjava+ribbon http://localhost:1051/um/getUserAndMovieUseRibbon?id=1 hystrix无效
ribbon http://localhost:1051/um/getAllUserRibbon hystrix有效
ribbon http://localhost:1051/um/getUserAndMovieUseRibbon1?id=1 hystrix有效