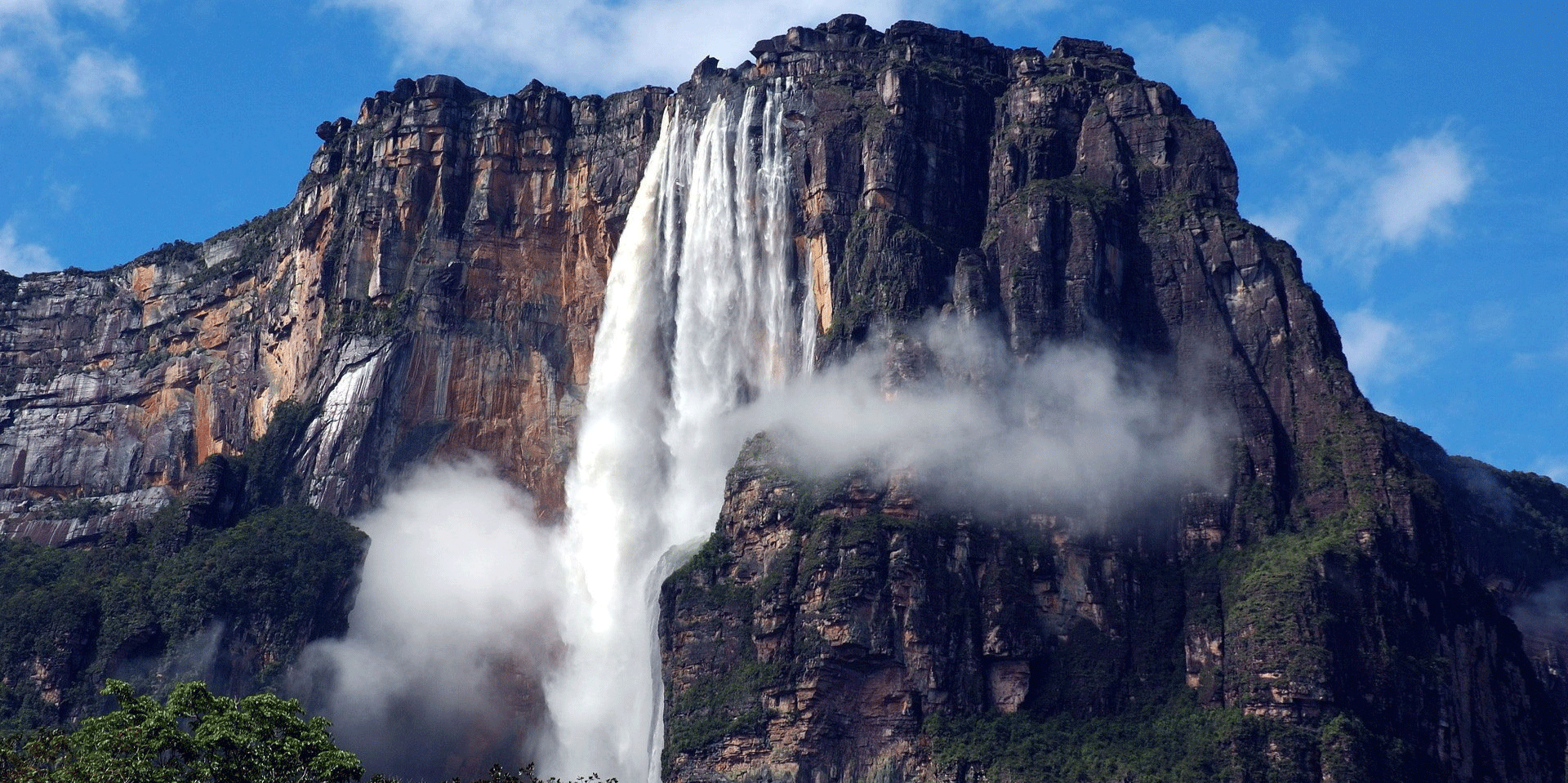
正文
添加依赖
maven
1 | <parent> |
添加配置
application.properties中添加如下配置
1 | spring.cache.type=jcache |
resources文件夹下面增加文件ehcache.xml,内容如下
1 | <config |
注意:以上ehcache配置表示支持持久化,在写入内存得时候同样会写入文件,重启后数据再次写入内存
编写配置类和服务类
如下是根据上面ehcache.xml中配置得所编写配置类和服务类
配置类
1 | package com.utstar.ucloud.springcloud.ucloudmpc.conf; |
服务类
1 | package com.utstar.filemonitoring.service; |
编写测试类测试上面服务类
1 | package com.utstar.filemonitoring.test; |
mockbean的这些类中的逻辑是springboot启动后需要执行的并且比较耗时,可以利用mockbean注入一个空类
1 | package com.utstar.filemonitoring.test.service; |