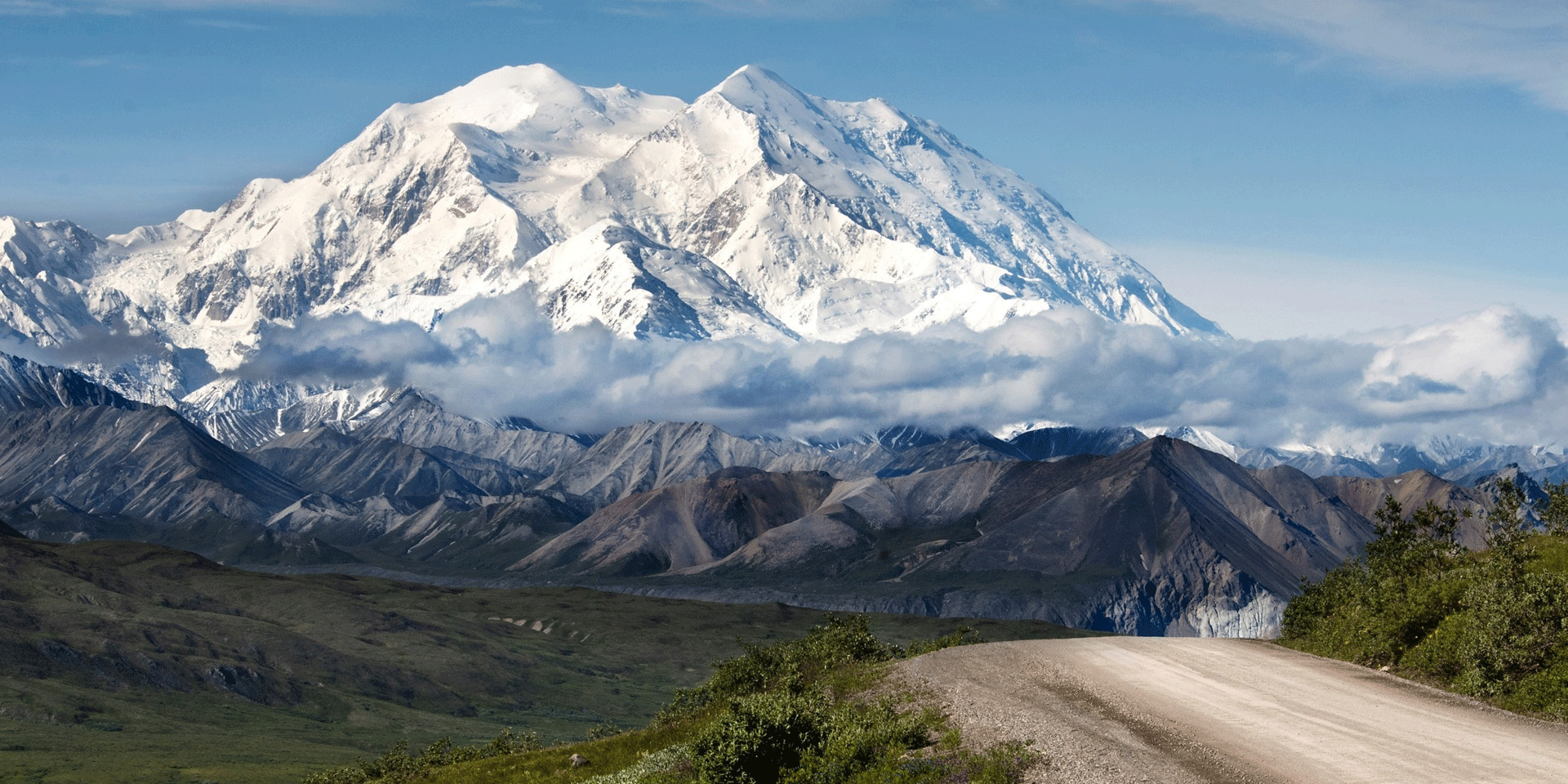
正文
添加依赖
maven 添加如下依赖
1 | <parent> |
添加配置
application.properties 配置文件添加如下配置
1 | netty.host=0.0.0.0 |
添加配置类和启动服务类
1 | package com.utstar.filemonitoring.netty; |
java后台技术栈
maven 添加如下依赖
1 | <parent> |
application.properties 配置文件添加如下配置
1 | netty.host=0.0.0.0 |
1 | package com.utstar.filemonitoring.netty; |