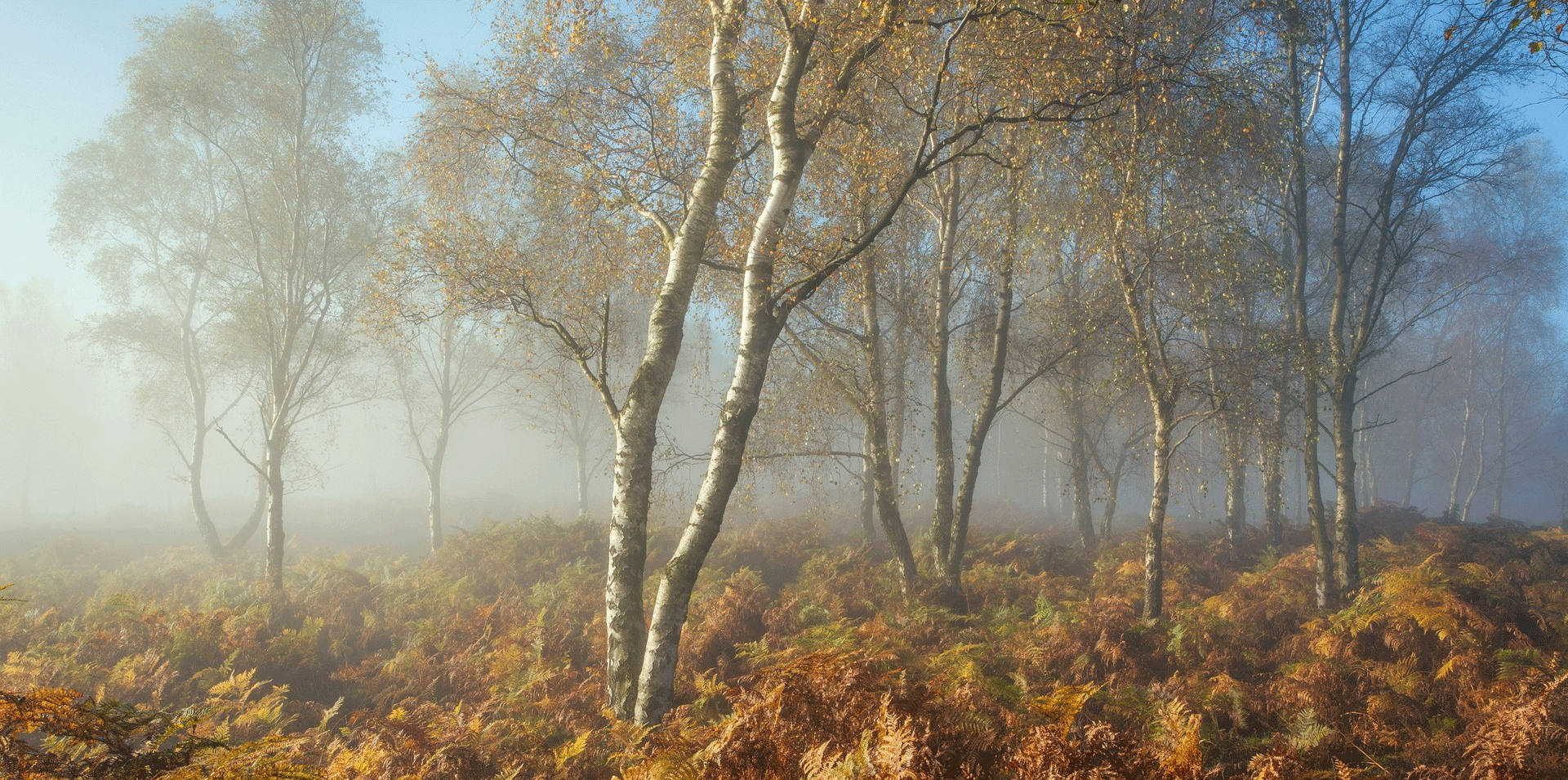
正文
异或(XOR)加密
原理
一个整数 a 和任意一个整数 b 异或两次,得到的结果是整数 a 本身,即: a == a ^ b ^ b
这里的 a 就是需要加密的原数据,b 则是密钥.a ^ b 就是加密过程,异或的结果就是加密后的密文
密文 (a ^ b) 再与密钥 b 异或,就是解密过程,得到的结果就是原数据 a 本身
源码
1 | package com.utstar.filemonitoring.utils; |
调用方式
1 | public static void main(String[] args) { |
DES加密
源码
1 | package com.creambing.utils.encrypt; |
由于上面用了lombok注解来调用日志,所以需要添加如下依赖
1 | <lombock.version>1.18.8</lombock.version> |
调用方式
1 | package com.creambing.utils.encrypt; |
上面加密的结果于下面网站一样
https://tool.lami.fun/jiami/des
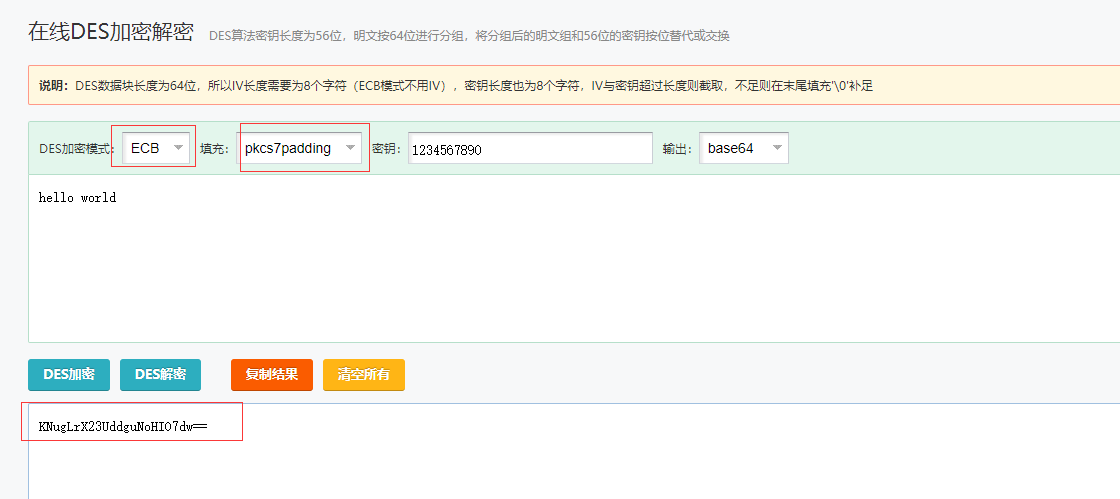